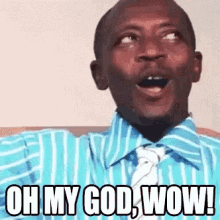
Lets Create a Recursive React Component for our File Explorer or Folders.
Lets Get a Sample JavaScript Variable for our Filesystem first. You can use API data or import a JSON if you wish to.
let Files = {
type: "folder",
name: "parent",
data: [
{
type: "folder",
name: "root",
data: [
{
type: "folder",
name: "src",
data: [
{
type: "file",
name: "index.js",
},
],
},
{
type: "folder",
name: "public",
data: [
{
type: "file",
name: "index.ts",
},
],
},
{
type: "file",
name: "index.html",
},
{
type: "folder",
name: "data",
data: [
{
type: "folder",
name: "images",
data: [
{
type: "file",
name: "image.png",
},
{
type: "file",
name: "image2.webp",
},
],
},
{
type: "file",
name: "logo.svg",
},
],
},
{
type: "file",
name: "style.css",
},
],
},
],
};
Alright so we got the files. Next we need to create a Component for our files.
function FileExplorer({ files }) {
const [expanded, setExpanded] = useState(false);
if (files.type === "folder") {
return (
<div key={files.name}>
<span onClick={() => setExpanded(!expanded)}>
{files.name}📂
</span>
<div
className="expanded"
style=
>
{files.data.map((file) => {
if (file.type === "file")
return <div key={file.name}>{file.name}</div>;
else if (file.type === "folder")
return <FileExplorer key={file.name} files={file} />;
})}
</div>
</div>
);
} else if (files.type === "file") {
return <div>{files.name}</div>;
}
}
export default function App() {
return (
<div className="App">
<FileExplorer files={Files} />
</div>
);
}
All we are trying to do here is simply is.
- Pass the File JSON to our Component as props.
- Inside the component check if the file passed is a folder or a file
- If its a file just render it and do nothing
- But if its a folder 📁📁 that's when the fun part begins.
- If its a folder then first show the label of the folder and below it render the
data
part of the folder. Here to toggle the data part we are using state in our component called asexpanded
. - Now comes the part when we need the recursion. Now while we are rendering the data we just need to check if the current file is a folder and if it is then simple call the
<FileExplorer/>
Component with the corresponding folder in the data. - And thats how it is done.
Check out this Codesandbox Example
I hope you learned something new today if you did then please share this post with your friends who might find this useful aswell. Have any questions? Feel free to connect with me on LinkedIn Twitter @singhkunal2050. You can also write me here.
Happy Coding 👩💻!