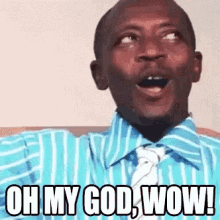
What is Fluid Typography?
When your font size changes according to the size of the window then we call it as a fluid typography.
There are several ways to make your fonts fluid across all resolutions in your websites. Here are 5 ways that I use regularly in my projects with examples.
- Good old media queries
- Using Relative Units vw, vh, Percentage (%)
- clamp() & calc()
- Using CSS Variables
Enough talk lets see the code
You can check this codepen for live example of all the points covered in this blog
See the Pen Responsive Typography CSS by Kunal SIngh (@singhkunal2050) on CodePen.
Good Old Media Queries @media-queries
media-queries
may not be the most fluid of all these methods since they are based on changing the size based on breakpoints of window
but they do come in handy and get the job done most of the time.
/* 1. With Media Queries */
.media h2{
font-size:2rem;
}
.media p{
font-size:1.2rem;
}
/* Setting breakpoint for changing font size */
@media (max-width:768px) {
.media h2{
font-size:1.5rem;
}
.media p{
font-size:.8rem;
}
}
Using Relative Units vw
vh
and %
This method uses relative units like percentage, vw and vh, we can set the font size on the basis of parent container(with %) or we can use the vw/vh unit which sets the sizes relative to the window. Although this method gives a completely fluid typography it has some pitfalls which we'll cover in the next point.
/* Using Relative % Units */
.relative-units h2{
font-size:5vw;
}
.relative-units p{
font-size:2.5vw;
}
Using CSS funtions clamp()
and calc()
The most annoying part of using a relative unit is we can not set any upper/lower limit to the values that we want. This problem is solved with the clamp()
function and the calc()
function comes with its own advantage of allowing us to do mathematic operations with our units like 10vw + 10px , 40%+ 2rem etc
/* Using clamp() , calc() & minmax() */
/* When this method is combined with relative units it gives the best results */
.css-functions h2{
font-size:calc(1.8rem + 2vw);
/* gives min/max size 👇 */
font-size:clamp(1.8rem, 5vw , 2.5rem );
}
.css-functions p{
font-size:clamp(.8rem, 4vw , 1.5rem );
/* does not give min/max size 👇 */
font-size:calc(.7rem + 1vw);
}
Using CSS Variables
Remember how we used media queries to swap font sizes on the basis of window
width? Using the same technique with css variables gives you the same feature with a little better code maintance option. Lets see how.
/* Using CSS Variables */
/* Setting Up Default Font size Variables */
:root{
--heading-2:2rem;
--content:1rem;
}
/* Overidding Variables with Media Queries */
@media(max-width:768px){
:root{
--heading-2:1.54rem;
--content:.7rem;
}
}
.css-var h2{
font-size:var(--heading-2);
}
.css-var p{
font-size:var(--content);
}
I hope you learned something new today if you did then please share this post with your friends who might find this useful aswell. Have any questions? Feel free to connect with me on LinkedIn Twitter @singhkunal2050. You can also write me here.
Happy Coding 👩💻!